Java工具類之@RequestMapping註解
一、前言
問題闡述:在某一場景下,我們的代碼在 Service 實現相同,但卻在 Controller 層訪問時卻希望不同的前綴可以訪問。如下 :/say/hello。我們這裡希望在不借助任何外部服務的情況下 通過 /a/say/hello 和 /b/say/hello 都可以訪問到該接口,同時不想在 Controller 中寫兩個方法。
@RestController @RequestMapping("say") public class SayController { @Autowired private SayService sayService; @RequestMapping("hello") public String hello() { return sayService.hello(); } }
二、代碼實現
我們這裡簡單說明一下思路:
1.在 Spring 服務啟動後, HandlerMapping 的實現類 RequestMappingHandlerMapping
會獲取到被 @RequestMapping等請求註解修飾的方法,並封裝成一個個 HandlerMethod 保存到 RequestMappingHandlerMapping#MappingRegistry
中(HandlerMapping 具有多個實現類,每個實現類具有不同規則)。
2.當 DispatcherServlet 接收到請求後會根據 url 獲取 合適的 HandlerMapping 組成 HandlerExecutionChain(處理器執行鏈),隨後通過 HandlerAdapter 來進行請求處理。而這裡通過 HandlerMapping 會根據請求 URL 獲取到匹配的 HandlerMethod 進行方法調用。
因此我們這裡有瞭兩種思路 :
1.在 Spring 加載 HandlerMethod 時設置當前 HandlerMethod 的匹配規則為 /a/say/hello/、/b/say/hello/,當 /a/say/hello/、/b/say/hello/ 請求訪問時可以與之匹配。
2.在請求處理的時候,通過攔截器將 /a/say/hello/、/b/say/hello/ 的訪問路徑匹配到 /say/hello 方法上。
本文選擇第一種思路(不過話說怎麼想都是第一種好吧)做一個簡單demo示例,其實現如下:
// 自定義分發註解 @Target({ElementType.TYPE, ElementType.METHOD}) @Retention(RetentionPolicy.RUNTIME) @Documented public @interface RequestRouter { String[] value() default ""; }
package com.kingfish.springjdbcdemo.config; import lombok.SneakyThrows; import org.springframework.stereotype.Component; import org.springframework.web.method.HandlerMethod; import org.springframework.web.servlet.mvc.condition.PatternsRequestCondition; import org.springframework.web.servlet.mvc.method.RequestMappingInfo; import org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping; import javax.servlet.http.HttpServletRequest; import java.lang.reflect.Field; import java.lang.reflect.Method; import java.util.Arrays; import java.util.Set; import java.util.stream.Collectors; /** * @Author : kingfish * @Email : [email protected] * @Data : 2021/4/21 16:47 * @Desc : 路由 HandlerMapping 的實現 */ @Component("handlerMapping") public class RouterRequestMappingHandlerMapping extends RequestMappingHandlerMapping { // 在將 方法封裝成 HandlerMethod 時會調用此方法 @SneakyThrows @Override protected RequestMappingInfo getMappingForMethod(Method method, Class<?> handlerType) { // 獲取 RequestRouter 註解 RequestRouter requestRouter = method.getAnnotation(RequestRouter.class); if (requestRouter == null) { requestRouter = handlerType.getAnnotation(RequestRouter.class); if (requestRouter == null) { for (Class<?> handlerTypeInterface : handlerType.getInterfaces()) { if ((requestRouter = handlerTypeInterface.getAnnotation(RequestRouter.class)) != null) { break; } } } } // 調用父類,生成 RequestMappingInfo RequestMappingInfo mappingForMethod = super.getMappingForMethod(method, handlerType); if (requestRouter != null) { // 如果 requestRouter 不為空,則進行路徑處理 String[] requestRouterValue = requestRouter.value(); PatternsRequestCondition condition = mappingForMethod.getPatternsCondition(); // 獲取當前方法匹配的路徑,隨即進行添加處理。 Set<String> patterns = condition.getPatterns(); Set<String> routerPatterns = patterns.stream() // 拼接 請求路徑。這裡可以自定義處理策略 .flatMap(pattern -> Arrays.stream(requestRouterValue).map(val -> "/" + val + pattern)) .collect(Collectors.toSet()); // 將拼接後的路徑添加到 RequestMappingInfo 中 patterns.addAll(routerPatterns); } return mappingForMethod; } }
@Configuration public class SpringConfig { @Bean public DispatcherServlet dispatcherServlet(){ DispatcherServlet dispatcherServlet = new DispatcherServlet(); // 禁止加載所有的handlerMapper,而隻加載beanName 為 handlerMapper 的bean dispatcherServlet.setDetectAllHandlerMappings(false); return dispatcherServlet; } }
這裡需要註意 :
1.HandlerMapping 在 Spring中有多個實現,而 dispatcherServlet.setDetectAllHandlerMappings(false);
參數設置Spring 放棄加載多個 HandlerMapping,而隻加載 beanName為 handlerMapping 的
2.HandlerMapping。RequestMappingInfo 包含 當前方法的諸多信息,其中就包含 什麼樣請求路徑可以匹配到該方法,所以我們在這裡獲取到 RequestRouter 的信息,並添加到匹配路徑上。
三、效果
在 方法上加上 @RequestRouter(value = {"a", "b"})
註解
@RestController @RequestMapping("say") public class SayController { @Autowired private SayService sayService; @RequestRouter(value = {"a", "b"}) @RequestMapping("hello") public String hello() { return sayService.hello(); } }
/a/say/hello/
、/b/say/hello/
以及 /say/hello/
都可以訪問
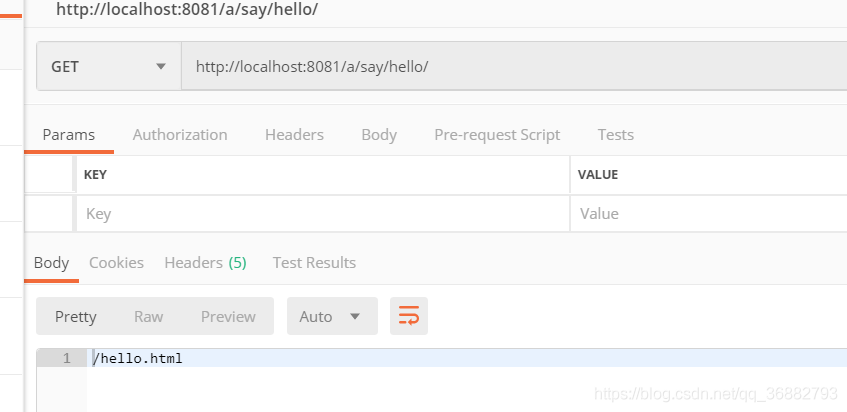
到此這篇關於Java工具類之@RequestMapping註解的文章就介紹到這瞭,更多相關Java RequestMapping內容請搜索WalkonNet以前的文章或繼續瀏覽下面的相關文章希望大傢以後多多支持WalkonNet!
推薦閱讀:
- SpringBoot根據目錄結構自動配置Url前綴方式
- SpringBoot根據目錄結構自動生成路由前綴的實現代碼
- Java中Controller引起的Ambiguous mapping問題及解決
- Springboot項目如何獲取所有的接口
- SpringBoot如何根據目錄路徑生成接口的url路徑