Java集合和IO流實現水果攤項目
前言
前一段時間利用Java基礎知識集合和IO流做瞭個簡單的小項目-水果攤,感覺不過癮,最近又想著用GUI和Mysql數據庫重做一下,名為另一個水果攤,下面就來分享一下代碼吧
一、包和表截圖
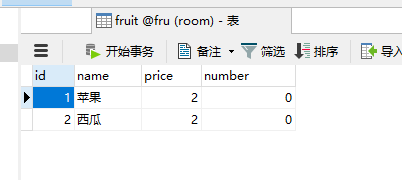
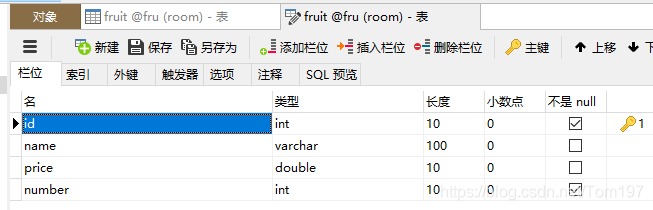
二、源代碼
1.JDBC連接Mysql數據
管理員界面:增刪查改
package com.vector.service; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import com.vector.dao.Fruit; import com.vector.units.ConnectMsql; public class FruitDao { public static String add(Fruit fruit) { String s = null; try { // Connection connection = ConnectMsql.getConnectMsql(); String sql = "insert into fruit(name,price,number) values(?,?,0)"; Connection connection = ConnectMsql.getConnectMsql(); PreparedStatement ad = connection.prepareStatement(sql); Statement stmt = connection.createStatement(); ResultSet rs = stmt.executeQuery("select * from fruit"); int f = 0; while (rs.next()) { if (fruit.getName().equals(rs.getString("name"))) { f = 1; } /* * System.out.println(rs.getInt("id") + " " + rs.getString("name") + " " + * rs.getDouble("price") + " " + rs.getInt("number")); */ } if (f == 0) { ad.setString(1, fruit.getName()); ad.setDouble(2, fruit.getPrice()); int i = ad.executeUpdate(); if (i > 0) { // System.out.println("添加成功!"); s = "添加成功!"; } else { // System.out.println("水果重復,添加失敗!"); s = "水果重復,添加失敗!"; } } else { s = "水果重復,添加失敗!"; } } catch (SQLException e) { // TODO Auto-generated catch block // e.printStackTrace(); } return s; } public static String delete(String name) { String sql = "delete from fruit where name = ?"; String s1 = null; Connection connection = ConnectMsql.getConnectMsql(); PreparedStatement dele = null; try { dele = connection.prepareStatement(sql); dele.setString(1, name); int i = dele.executeUpdate(); if (i > 0) { s1 = "刪除成功!"; } else { s1 = "刪除失敗!未找到該水果!"; } } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } return s1; } public static String change(String name, double price) { String sql = "update fruit set price= ? where name= ?"; String s2 = null; try { Connection connection = ConnectMsql.getConnectMsql(); PreparedStatement upda = connection.prepareStatement(sql); upda.setDouble(1, price); // upda.setInt(2, 2); upda.setString(2, name); // System.out.println(name); int i = upda.executeUpdate(); if (i > 0) { s2 = " 修改成功!"; } else { s2 = "修改失敗,水果不存在!"; } } catch (SQLException e) { e.printStackTrace(); } return s2; } public static ResultSet list() { ResultSet rs = null; Connection connection = ConnectMsql.getConnectMsql(); Statement stmt; try { stmt = connection.createStatement(); rs = stmt.executeQuery("select * from fruit"); /* * while (rs.next()) { s="序號:"+rs.getInt("id") + " 水果名稱:" + rs.getString("name") * + " 水果價格:" + rs.getDouble("price") + " "; * System.out.println("序號:"+rs.getInt("id") + " 水果名稱:" + rs.getString("name") + * " 水果價格:" + rs.getDouble("price") + " " ); * * } */ } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } return rs; } }
用戶界面 :查找水果
package com.vector.service; import java.sql.Connection; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import java.util.ArrayList; import java.util.List; import com.vector.dao.Fruit; import com.vector.units.ConnectMsql; public class UFruitDao { public static List<Fruit> list = new ArrayList<>(); static Fruit f = null; public static List Uadd(String name, int number) { ResultSet rs = null; Connection connection = ConnectMsql.getConnectMsql(); try { Statement stmt = connection.createStatement(); rs = stmt.executeQuery("select * from fruit"); int k = 0; while (rs.next()) { if (rs.getString("name").equals(name)) { f = new Fruit(rs.getString("name"), rs.getDouble("price"), number); k = 1; } /* * System.out.println("序號:" + rs.getInt("id") + " 水果名稱:" + rs.getString("name") * + " 水果價格:" + rs.getDouble("price") + " "); */ } if (k == 0) { System.out.println("水果不純在,添加失敗!"); } k = 0; for (Fruit s : list) { if (s.getName().equals(name)) { s.setNumber(s.getNumber() + number); k = 1; } } if (k == 0) list.add(f); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } return list; } }
建立斷開連接
package com.vector.units; import java.sql.*; public class ConnectMsql { public static Connection connect; public static Connection getConnectMsql() { try { Class.forName("com.mysql.cj.jdbc.Driver"); // Class.forName("org.gjt.mm.mysql.Driver"); // System.out.println("成功加載Mysql驅動程序!"); } catch (Exception e) { System.out.print("加載Mysql驅動程序時出錯!"); e.printStackTrace(); } try { connect = DriverManager.getConnection( "jdbc:mysql://localhost:3306/fru?&useSSL=false&serverTimezone=UTC&allowPublicKeyRetrieval=true", "root", "zpx"); // System.out.println("成功連接Mysql服務器!"); Statement stmt = connect.createStatement(); } catch (Exception e) { System.out.print("獲取連接錯誤!"); e.printStackTrace(); } return connect; } public static void closeConnection() { if (connect != null) { try { connect.close(); // sSystem.out.println("數據庫連接關閉"); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } }
2.GUI窗口界面
開始選擇界面
package com.vector.view; import java.awt.BorderLayout; import java.awt.EventQueue; import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.border.EmptyBorder; import com.vector.test.logon; import com.vector.view.userInterface; import javax.swing.JMenuBar; import javax.swing.JMenu; import javax.swing.JMenuItem; import java.awt.Toolkit; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.ImageIcon; import javax.swing.JTextArea; import java.awt.Color; import java.awt.CardLayout; import javax.swing.JLabel; import javax.swing.SwingConstants; import java.awt.SystemColor; public class selInterface extends JFrame { private JPanel contentPane; /** * Launch the application. */ public static void star() { EventQueue.invokeLater(new Runnable() { public void run() { try { selInterface frame = new selInterface(); frame.setVisible(true); } catch (Exception e) { e.printStackTrace(); } } }); } /** * Create the frame. */ public selInterface() { setIconImage(Toolkit.getDefaultToolkit().getImage("D:\\eclip\\FruitV\\src\\tubiao.png")); //左上角圖標 setTitle("\u6C34\u679C\u644A"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setBounds(100, 100, 600, 600); JMenuBar menuBar = new JMenuBar(); menuBar.setToolTipText(""); setJMenuBar(menuBar); JMenu menu = new JMenu("\u9875\u9762\u9009\u62E9"); menuBar.add(menu); Monitor2 monitor = new Monitor2(); JMenu menu_1 = new JMenu("\u7BA1\u7406\u5458\u754C\u9762"); menu_1.setIcon(new ImageIcon("D:\\eclip\\FruitV\\src\\556.png")); //管理員圖標 menu.add(menu_1); JMenuItem menuItem = new JMenuItem("\u767B\u5F55"); menu_1.add(menuItem); menuItem.addActionListener(monitor); JMenuItem menuItem_2 = new JMenuItem("\u987E\u5BA2\u754C\u9762"); menuItem_2.setIcon(new ImageIcon("D:\\eclip\\FruitV\\src\\557.png")); //登錄圖標 menu.add(menuItem_2); menuItem_2.addActionListener(monitor); contentPane = new JPanel(); contentPane.setBackground(Color.WHITE); contentPane.setBorder(new EmptyBorder(5, 5, 5, 5)); setContentPane(contentPane); contentPane.setLayout(null); JLabel lblNewLabel_1 = new JLabel(""); lblNewLabel_1.setBounds(0, 71, 584, 465); lblNewLabel_1.setIcon(new ImageIcon("D:\\eclip\\FruitV\\src\\122.png")); //顧客圖標 contentPane.add(lblNewLabel_1); JLabel label = new JLabel("\u6B22\u8FCE\u6765\u5230\u6C34\u679C\u644A"); label.setBounds(245, 0, 99, 71); contentPane.add(label); JLabel lblNewLabel = new JLabel(""); lblNewLabel.setIcon(new ImageIcon("D:\\eclip\\FruitV\\src\\lan.png")); //窗口上方藍色方塊 lblNewLabel.setBackground(Color.GREEN); lblNewLabel.setBounds(0, 0, 584, 71); contentPane.add(lblNewLabel); ImageIcon ig = new ImageIcon("D:\\eclip\\FruitV\\src\\tubiao.png"); //左上角圖標 ImageIcon img = new ImageIcon("D:\\eclip\\FruitV\\src\\QQ圖片20210420205633.png"); //窗口背景圖 JLabel imgLabel = new JLabel(img); this.getLayeredPane().add(imgLabel, new Integer(Integer.MIN_VALUE)); imgLabel.setBounds(0, 0, img.getIconWidth(), img.getIconHeight()); } } class Monitor2 implements ActionListener { public void actionPerformed(ActionEvent e) { //System.out.println("有響應"); String buttonName = e.getActionCommand(); if(buttonName.equals("登錄")) { logon.star(); } if(buttonName.equals("顧客界面")) { System.out.println("顧客界面"); userInterface.start(); } } }
用戶購買水果界面
package com.vector.view; import java.awt.Image; import java.awt.List; import java.awt.Toolkit; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.util.ArrayList; import java.util.jar.JarFile; import javax.swing.*; import com.vector.dao.Fruit; import com.vector.service.UFruitDao; public class userInterface extends JFrame { public static ArrayList <Fruit> list; static double x; JPanel contentPane; Image im; Box boxH, boxH1; Box boxVOne, boxVTwo; Box button, show; Box show1, show2; public static JTextArea a1,a2; public userInterface() { setLayout(new java.awt.FlowLayout()); setResizable(false); contentPane = new JPanel(); setContentPane(contentPane); contentPane.setOpaque(false); setVisible(true); setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE); init(); } void init() { ImageIcon ig = new ImageIcon("D:\\eclip\\FruitV\\src\\tubiao.png"); im = ig.getImage(); setIconImage(im); ImageIcon img = new ImageIcon("D:\\eclip\\FruitV\\src\\beijing.png"); JLabel imgLabel = new JLabel(img); this.getLayeredPane().add(imgLabel, new Integer(Integer.MIN_VALUE)); imgLabel.setBounds(0, 0, img.getIconWidth(), img.getIconHeight()); boxH = Box.createHorizontalBox(); boxVOne = Box.createVerticalBox(); boxVTwo = Box.createVerticalBox(); button = Box.createHorizontalBox(); show = Box.createVerticalBox(); show1 = Box.createVerticalBox(); show2 = Box.createVerticalBox(); boxH1 = Box.createHorizontalBox(); JTextField t1 = new JTextField(10); JTextField t2 = new JTextField(10); JTextField t3 = new JTextField(10); a1 = new JTextArea(9, 30); a2 = new JTextArea(4, 30); JScrollPane s1=new JScrollPane(a1); JScrollPane s2=new JScrollPane(a2); JButton b1 = new JButton("確定添加"); JButton b2 = new JButton("確定購買"); JButton b3 = new JButton("確定付款"); boxVOne.add(new JLabel("水果名稱:")); boxVOne.add(new JLabel("水果數量(斤):")); boxVTwo.add(t1); boxVTwo.add(t2); button.add(b1); show.add(s1); show.add(Box.createVerticalStrut(20)); show.add(Box.createHorizontalStrut(110)); show.add(b2); show.add(Box.createVerticalStrut(20)); boxH.add(boxVOne); boxH.add(Box.createHorizontalStrut(10)); boxH.add(boxVTwo); boxH.add(button); add(boxH); add(show); boxH1.add(new JLabel("請付款:")); boxH1.add(Box.createHorizontalStrut(10)); boxH1.add(t3); boxH1.add(b3); show2.add(boxH1); show2.add(Box.createVerticalStrut(10)); show2.add(s2); add(show1); add(show2); Monitor1 monitor =new Monitor1(); b1.addActionListener(monitor); b2.addActionListener(monitor); b3.addActionListener(monitor); monitor.setText1(t1); monitor.setText2(t2); monitor.setText3(t3); } public class Monitor1 implements ActionListener { JTextField text1,text2,text3; public void setText1(JTextField text1) { this.text1 = text1; } public void setText2(JTextField text2) { this.text2 = text2; } public void setText3(JTextField text3) { this.text3 = text3; } @Override public void actionPerformed(ActionEvent e) { // TODO Auto-generated method stub String buttonName = e.getActionCommand(); try { if(buttonName.equals("確定添加")) { String name=text1.getText(); String number0 = (String) text2.getText(); int number = Integer.valueOf(number0.toString()); list =new ArrayList<>(); list = (ArrayList<Fruit>) UFruitDao.Uadd(name, number); a1.setText(""); for (Fruit s : list) { //System.out.println(s.toString()); a1.append(s.toString()+"\n"); } System.out.println("添加成功!"); } if(buttonName.equals("確定購買")) { x=0; for (Fruit s : list) { x+=s.getNumber()*s.getPrice(); } a1.append("請付款:"+x+"元。"+"\n"); } if(buttonName.equals("確定付款")) { String money0 = (String) text3.getText(); double money = Double.valueOf(money0.toString()); double s=money-x; a2.setText(""); if(s>0) { //System.out.println("付款成功,找你"+s+"元。"); a2.append("付款成功,找你"+s+"元,慢走不送!"); } if(s==0) { //System.out.println("付款成功,剛好夠"); a2.append("付款成功,剛好夠,慢走不送!"); } if(s<0) { //System.out.println("付款失敗,錢不夠"); a2.append("付款失敗,錢不夠。"); } } }catch(Exception e2) { System.out.println("添加失敗,請按要求輸入!"); } } } public static void start() { userInterface win = new userInterface(); win.setBounds(100, 100, 600, 600); win.setTitle("水果攤"); } }
管理員登錄界面
package com.vector.test; import java.awt.BorderLayout; import java.awt.EventQueue; import javax.swing.JFrame; import javax.swing.JPanel; import javax.swing.border.EmptyBorder; import com.vector.view.admInterface; import javax.swing.JLabel; import java.awt.Color; import javax.swing.JTextField; import javax.swing.JPasswordField; import javax.swing.JButton; import javax.swing.ImageIcon; import java.awt.Toolkit; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; public class logon extends JFrame { private JPanel contentPane; private JTextField textField; private JPasswordField passwordField; /** * Launch the application. */ public static void star() { EventQueue.invokeLater(new Runnable() { public void run() { try { logon frame = new logon(); frame.setVisible(true); } catch (Exception e) { e.printStackTrace(); } } }); } /** * Create the frame. */ public logon() { setIconImage(Toolkit.getDefaultToolkit().getImage("D:\\eclip\\FruitV\\src\\556.png")); setTitle("\u7BA1\u7406\u5458\u767B\u5F55\u7CFB\u7EDF"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setBounds(100, 100, 600, 600); contentPane = new JPanel(); contentPane.setBorder(new EmptyBorder(5, 5, 5, 5)); setContentPane(contentPane); contentPane.setLayout(null); JLabel label = new JLabel("\u7BA1\u7406\u5458\u767B\u5F55\u7CFB\u7EDF"); label.setForeground(new Color(139, 0, 139)); label.setBounds(239, 38, 112, 15); contentPane.add(label); JLabel label_1 = new JLabel("\u7528\u6237\u540D\uFF1A"); label_1.setBounds(120, 98, 54, 15); contentPane.add(label_1); textField = new JTextField(); textField.setText("1+1=\uFF1F"); textField.setBounds(179, 95, 214, 21); contentPane.add(textField); textField.setColumns(10); JLabel label_2 = new JLabel("\u5BC6\u7801\uFF1A"); label_2.setBounds(120, 162, 54, 15); contentPane.add(label_2); Monitor3 monitor =new Monitor3(); passwordField = new JPasswordField(); passwordField.setBounds(179, 159, 214, 18); contentPane.add(passwordField); monitor.setP1(passwordField); JButton button = new JButton("\u767B\u5F55"); button.setBounds(193, 228, 93, 23); contentPane.add(button); button.addActionListener(monitor); JButton button_1 = new JButton("\u9000\u51FA"); button_1.setBounds(296, 228, 93, 23); contentPane.add(button_1); button_1.addActionListener(monitor); JLabel label_4 = new JLabel("\u731C\u731C\u5BC6\u7801\u662F\u4EC0\u4E48......"); label_4.setForeground(new Color(139, 0, 0)); label_4.setBounds(231, 341, 176, 15); contentPane.add(label_4); JLabel lblNewLabel = new JLabel(""); lblNewLabel.setIcon(new ImageIcon("D:\\eclip\\FruitV\\src\\dongtai.gif")); lblNewLabel.setBounds(231, 379, 123, 99); contentPane.add(lblNewLabel); JLabel lblNewLabel_1 = new JLabel(""); lblNewLabel_1.setBounds(401, 162, 106, 15); contentPane.add(lblNewLabel_1); } } class Monitor3 implements ActionListener { JPasswordField p1; public void setP1(JPasswordField p1) { this.p1 = p1; } public void actionPerformed(ActionEvent e) { String s=p1.getText(); String buttname=e.getActionCommand(); if(buttname.equals("登錄")) { if(s.equals("2")) { admInterface.start(); }else { System.out.println("密碼錯誤!"); } } if(buttname.equals("退出")) { System.exit(0); } } }
管理員界面
package com.vector.view; import java.awt.Component; import java.awt.FlowLayout; import java.awt.Image; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.sql.ResultSet; import java.sql.SQLException; import javax.swing.Box; import javax.swing.ImageIcon; import javax.swing.JButton; import javax.swing.JComboBox; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JScrollPane; import javax.swing.JTextArea; import javax.swing.JTextField; import com.vector.dao.Fruit; import com.vector.service.FruitDao; import com.vector.units.ConnectMsql; public class admInterface extends JFrame { public static JTextArea a1; public static JTextArea a2; Image im; public static Fruit fruit; public admInterface() { setLayout(new FlowLayout()); setVisible(true); setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE); ImageIcon ig = new ImageIcon("D:\\eclip\\FruitV\\src\\tubiao.png"); im = ig.getImage(); setIconImage(im); // Component H= Box.createHorizontalStrut(10); // Component V= Box.createVerticalStrut(10); Box boxH1 = Box.createHorizontalBox(); Box boxH2 = Box.createHorizontalBox(); Box boxH3 = Box.createHorizontalBox(); Box boxH4 = Box.createHorizontalBox(); Box boxH5 = Box.createHorizontalBox(); Box boxV = Box.createVerticalBox(); JTextField t1 = new JTextField(10); JTextField t2 = new JTextField(10); JTextField t3 = new JTextField(10); JTextField t4 = new JTextField(10); JTextField t5 = new JTextField(10); JButton B1 = new JButton("確定添加"); JButton B2 = new JButton("確定刪除"); JButton B3 = new JButton("確定修改"); JButton B4 = new JButton("刷新"); a1 = new JTextArea(8, 20); a2 = new JTextArea(11, 20); JScrollPane scroll1 = new JScrollPane(a1); JScrollPane scroll2 = new JScrollPane(a2); boxH1.add(new JLabel("水果名稱:")); boxH1.add(t1); boxH1.add(Box.createHorizontalStrut(10)); boxH1.add(new JLabel("水果價格(/斤):")); boxH1.add(t2); boxH1.add(Box.createHorizontalStrut(10)); boxH1.add(B1); boxH2.add(new JLabel("水果名稱:")); boxH2.add(Box.createHorizontalStrut(10)); boxH2.add(t3); boxH2.add(Box.createHorizontalStrut(10)); boxH2.add(B2); boxH3.add(new JLabel("水果名稱:")); boxH3.add(Box.createHorizontalStrut(10)); boxH3.add(t4); boxH3.add(Box.createHorizontalStrut(10)); boxH3.add(new JLabel("水果新價格(/斤):")); boxH3.add(Box.createHorizontalStrut(10)); boxH3.add(t5); boxH3.add(B3); boxH4.add(new JLabel("操作結果:")); boxH4.add(Box.createHorizontalStrut(10)); boxH4.add(scroll1); boxH5.add(new JLabel("水果列表:")); boxH5.add(Box.createHorizontalStrut(10)); boxH5.add(scroll2); boxV.add(Box.createVerticalStrut(10)); boxV.add(new JLabel("增加水果")); boxV.add(Box.createVerticalStrut(10)); boxV.add(boxH1); boxV.add(Box.createVerticalStrut(20)); boxV.add(new JLabel("刪除水果")); boxV.add(Box.createVerticalStrut(10)); boxV.add(boxH2); boxV.add(Box.createVerticalStrut(20)); boxV.add(new JLabel("更改水果價格")); boxV.add(Box.createVerticalStrut(10)); boxV.add(boxH3); boxV.add(Box.createVerticalStrut(20)); boxV.add(boxH4); boxV.add(Box.createVerticalStrut(20)); boxV.add(boxH5); boxV.add(Box.createVerticalStrut(10)); boxV.add(B4); add(boxV); Monitor monitor = new Monitor(); B1.addActionListener(monitor); B2.addActionListener(monitor); B3.addActionListener(monitor); B4.addActionListener(monitor); monitor.settext1(t1); monitor.settext2(t2); monitor.settext3(t3); monitor.settext4(t4); monitor.settext5(t5); } public class Monitor implements ActionListener { JTextField text1, text2, text3, text4, text5; public void settext1(JTextField str) { text1 = str; } public void settext2(JTextField str) { text2 = str; } public void settext3(JTextField str) { text3 = str; } public void settext4(JTextField str) { text4 = str; } public void settext5(JTextField str) { text5 = str; } public void actionPerformed(ActionEvent e) { String buttonName = e.getActionCommand(); try { if (buttonName.equals("確定添加")) { String name = text1.getText(); String price0 = (String) text2.getText(); double price = Double.valueOf(price0.toString()); // System.out.println(name + price); fruit = new Fruit(name, price, 0); String s = FruitDao.add(fruit); a1.append(s + "\n"); ConnectMsql.closeConnection(); } if (buttonName.equals("確定刪除")) { String name = text3.getText(); String s = null; try { s = FruitDao.delete(name); } catch (Exception e1) { // TODO Auto-generated catch block // e1.printStackTrace(); System.out.println("添加失敗,請輸入正確的格式!"); } a1.append(s + "\n"); ConnectMsql.closeConnection(); } if (buttonName.equals("確定修改")) { String name = text4.getText(); // System.out.println(name); String price0 = (String) text5.getText(); String s = null; double price = Double.valueOf(price0.toString()); try { s = FruitDao.change(name, price); } catch (Exception e2) { e2.printStackTrace(); } a1.append(s + "\n"); ConnectMsql.closeConnection(); } if (buttonName.equals("刷新")) { ResultSet rs = FruitDao.list(); try { a2.setText(""); while (rs.next()) { a2.append("序號:" + rs.getInt("id") + ", 水果名稱:" + rs.getString("name") + ", 水果價格:" + rs.getDouble("price") + " \n"); } } catch (SQLException e1) { // TODO Auto-generated catch block System.out.println("添加失敗,請輸入正確的格式!"); // e1.printStackTrace(); } ConnectMsql.closeConnection(); } } catch (Exception e1) { System.out.println("添加失敗,請依照正確格式輸入!"); a1.append("添加失敗,請依照正確格式輸入!"); } } } public static void start() { admInterface win = new admInterface(); win.setBounds(100, 100, 600, 700); win.setTitle("管理員頁面"); } }
3.水果屬性
package com.vector.dao; public class Fruit { private String name; private double price; private int number; public String getName() { return name; } public void setName(String name) { this.name = name; } public double getPrice() { return price; } public void setPrice(double price) { this.price = price; } public int getNumber() { return number; } public void setNumber(int number) { this.number = number; } public Fruit( String name, double price, int number) { this.name = name; this.price = price; this.number = number; } public String toString() { return "水果名稱:"+name+",水果價格:"+price+",購買水果數量:"+number; } }
4.main方法
package com.vector.domain; import com.vector.view.selInterface; public class mainV { public static void main(String[] args) { selInterface.star(); } }
到此這篇關於Java小項目另一個水果攤的文章就介紹到這瞭,更多相關Java水果攤內容請搜索WalkonNet以前的文章或繼續瀏覽下面的相關文章希望大傢以後多多支持WalkonNet!