SpringBoot與SpringSecurity整合方法附源碼
依賴
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- Thymeleaf --> <dependency> <groupId>org.thymeleaf</groupId> <artifactId>thymeleaf-spring5</artifactId> </dependency> <dependency> <groupId>org.thymeleaf.extras</groupId> <artifactId>thymeleaf-extras-java8time</artifactId> </dependency> <!-- SpringSecurity --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency> <!-- Thymeleaf 與 SpringSecurity 整合包 --> <dependency> <groupId>org.thymeleaf.extras</groupId> <artifactId>thymeleaf-extras-springsecurity5</artifactId> <version>3.0.4.RELEASE</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> <exclusions> <exclusion> <groupId>org.junit.vintage</groupId> <artifactId>junit-vintage-engine</artifactId> </exclusion> </exclusions> </dependency> </dependencies>
Controller:
package com.blu.controller; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; @Controller public class RouterController { @RequestMapping({ "/", "/index" }) public String index() { return "index"; } @RequestMapping("/tologin") public String toLogin() { return "views/login"; } @RequestMapping("/level1/{id}") public String level1(@PathVariable("id") int id) { return "views/level1/" + id; } @RequestMapping("/level2/{id}") public String level2(@PathVariable("id") int id) { return "views/level2/" + id; } @RequestMapping("/level3/{id}") public String level3(@PathVariable("id") int id) { return "views/level3/" + id; } }
SecurityConfig:
package com.blu.config; import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder; @EnableWebSecurity public class SecurityConfig extends WebSecurityConfigurerAdapter{ /** * 授權 */ @Override protected void configure(HttpSecurity http) throws Exception { //所有人可以訪問首頁,功能頁需要指定權限才可以訪問 http.authorizeRequests() .antMatchers("/").permitAll() .antMatchers("/level1/**").hasRole("vip1") .antMatchers("/level2/**").hasRole("vip2") .antMatchers("/level3/**").hasRole("vip3"); //沒有權限將默認跳轉至登錄頁,需要開啟登錄的頁面 //loginPage設置跳轉至登錄頁的請求(默認為/login) //usernameParameter和passwordParameter配置登錄的用戶名和密碼參數名稱,默認就是username和password //loginProcessingUrl配置登錄請求的url,需要和表單提交的url一致 http.formLogin().loginPage("/tologin") .usernameParameter("username") .passwordParameter("password") .loginProcessingUrl("/login"); //禁用CSRF保護 http.csrf().disable(); //開啟註銷功能和註銷成功後的跳轉頁面(默認為登錄頁面) http.logout().logoutSuccessUrl("/"); //開啟記住我功能,Cookie默認保存兩周 http.rememberMe().rememberMeParameter("remember"); } /** * 認證 */ @Override protected void configure(AuthenticationManagerBuilder auth) throws Exception { auth.inMemoryAuthentication().passwordEncoder(new BCryptPasswordEncoder()) .withUser("BLU").password(new BCryptPasswordEncoder().encode("123456")).roles("vip2","vip3") .and() .withUser("root").password(new BCryptPasswordEncoder().encode("111111")).roles("vip1","vip2","vip3") .and() .withUser("guest").password(new BCryptPasswordEncoder().encode("111222")).roles("vip1"); } }
註:以上方式認證的用戶和角色信息是存儲在內存中的,在實際開發中應該從數據庫中獲取,詳見:SpringSecurity從數據庫中獲取用戶信息進行驗證
index.html
<!DOCTYPE html> <html lang="en" xmlns:th="http://www.thymeleaf.org" xmlns:sec="http://www.thymeleaf.org/thymeleaf-extras-springsecurity5"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1"> <title>首頁</title> <!--semantic-ui--> <link href="https://cdn.bootcss.com/semantic-ui/2.4.1/semantic.min.css" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="stylesheet"> <link th:href="@{/qinjiang/css/qinstyle.css}" rel="external nofollow" rel="external nofollow" rel="stylesheet"> </head> <body> <!--主容器--> <div class="ui container"> <div class="ui segment" id="index-header-nav" th:fragment="nav-menu"> <div class="ui secondary menu"> <a class="item" th:href="@{/index}" rel="external nofollow" >首頁</a> <!--登錄註銷--> <div class="right menu"> <!--如果未登錄--> <div sec:authorize="!isAuthenticated()"> <a class="item" th:href="@{/tologin}" rel="external nofollow" > <i class="address card icon"></i> 登錄 </a> </div> <!--如果已登錄--> <div sec:authorize="isAuthenticated()"> <a class="item"> <i class="address card icon"></i> 用戶名:<span sec:authentication="principal.username"></span> 角色:<span sec:authentication="principal.authorities"></span> </a> </div> <div sec:authorize="isAuthenticated()"> <a class="item" th:href="@{/logout}" rel="external nofollow" > <i class="address card icon"></i> 註銷 </a> </div> </div> </div> </div> <div class="ui segment" style="text-align: center"> <h3>Spring Security Study by BLU</h3> </div> <div> <br> <div class="ui three column stackable grid"> <div class="column" sec:authorize="hasRole('vip1')"> <div class="ui raised segment"> <div class="ui"> <div class="content"> <h5 class="content">Level 1</h5> <hr> <div><a th:href="@{/level1/1}" rel="external nofollow" ><i class="bullhorn icon"></i> Level-1-1</a></div> <div><a th:href="@{/level1/2}" rel="external nofollow" ><i class="bullhorn icon"></i> Level-1-2</a></div> <div><a th:href="@{/level1/3}" rel="external nofollow" ><i class="bullhorn icon"></i> Level-1-3</a></div> </div> </div> </div> </div> <div class="column" sec:authorize="hasRole('vip2')"> <div class="ui raised segment"> <div class="ui"> <div class="content"> <h5 class="content">Level 2</h5> <hr> <div><a th:href="@{/level2/1}" rel="external nofollow" ><i class="bullhorn icon"></i> Level-2-1</a></div> <div><a th:href="@{/level2/2}" rel="external nofollow" ><i class="bullhorn icon"></i> Level-2-2</a></div> <div><a th:href="@{/level2/3}" rel="external nofollow" ><i class="bullhorn icon"></i> Level-2-3</a></div> </div> </div> </div> </div> <div class="column" sec:authorize="hasRole('vip3')"> <div class="ui raised segment"> <div class="ui"> <div class="content"> <h5 class="content">Level 3</h5> <hr> <div><a th:href="@{/level3/1}" rel="external nofollow" ><i class="bullhorn icon"></i> Level-3-1</a></div> <div><a th:href="@{/level3/2}" rel="external nofollow" ><i class="bullhorn icon"></i> Level-3-2</a></div> <div><a th:href="@{/level3/3}" rel="external nofollow" ><i class="bullhorn icon"></i> Level-3-3</a></div> </div> </div> </div> </div> </div> </div> </div> <script th:src="@{/qinjiang/js/jquery-3.1.1.min.js}"></script> <script th:src="@{/qinjiang/js/semantic.min.js}"></script> </body> </html>
views/login.html
<!DOCTYPE html> <html lang="en" xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1"> <title>登錄</title> <!--semantic-ui--> <link href="https://cdn.bootcss.com/semantic-ui/2.4.1/semantic.min.css" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="stylesheet"> </head> <body> <!--主容器--> <div class="ui container"> <div class="ui segment"> <div style="text-align: center"> <h1 class="header">登錄</h1> </div> <div class="ui placeholder segment"> <div class="ui column very relaxed stackable grid"> <div class="column"> <div class="ui form"> <form th:action="@{/login}" method="post"> <div class="field"> <label>Username</label> <div class="ui left icon input"> <input type="text" placeholder="Username" name="username"> <i class="user icon"></i> </div> </div> <div class="field"> <label>Password</label> <div class="ui left icon input"> <input type="password" name="password"> <i class="lock icon"></i> </div> </div> <div class="field"> <input type="checkbox" name="remember"> 記住我 </div> <input type="submit" class="ui blue submit button"/> </form> </div> </div> </div> </div> <div style="text-align: center"> <div class="ui label"> </i>註冊 </div> <br><br> <small>[email protected]</small> </div> <div class="ui segment" style="text-align: center"> <h3>Spring Security Study by BLU</h3> </div> </div> </div> <script th:src="@{/qinjiang/js/jquery-3.1.1.min.js}"></script> <script th:src="@{/qinjiang/js/semantic.min.js}"></script> </body> </html>
views/level1/1.html
<!DOCTYPE html> <html lang="en" xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1"> <title>首頁</title> <!--semantic-ui--> <link href="https://cdn.bootcss.com/semantic-ui/2.4.1/semantic.min.css" rel="external nofollow" rel="external nofollow" rel="external nofollow" rel="stylesheet"> <link th:href="@{/qinjiang/css/qinstyle.css}" rel="external nofollow" rel="external nofollow" rel="stylesheet"> </head> <body> <!--主容器--> <div class="ui container"> <div th:replace="~{index::nav-menu}"></div> <div class="ui segment" style="text-align: center"> <h3>Level-1-1</h3> </div> </div> <script th:src="@{/qinjiang/js/jquery-3.1.1.min.js}"></script> <script th:src="@{/qinjiang/js/semantic.min.js}"></script> </body> </html>
views/level2/1.html 等其他頁面:略
運行效果:
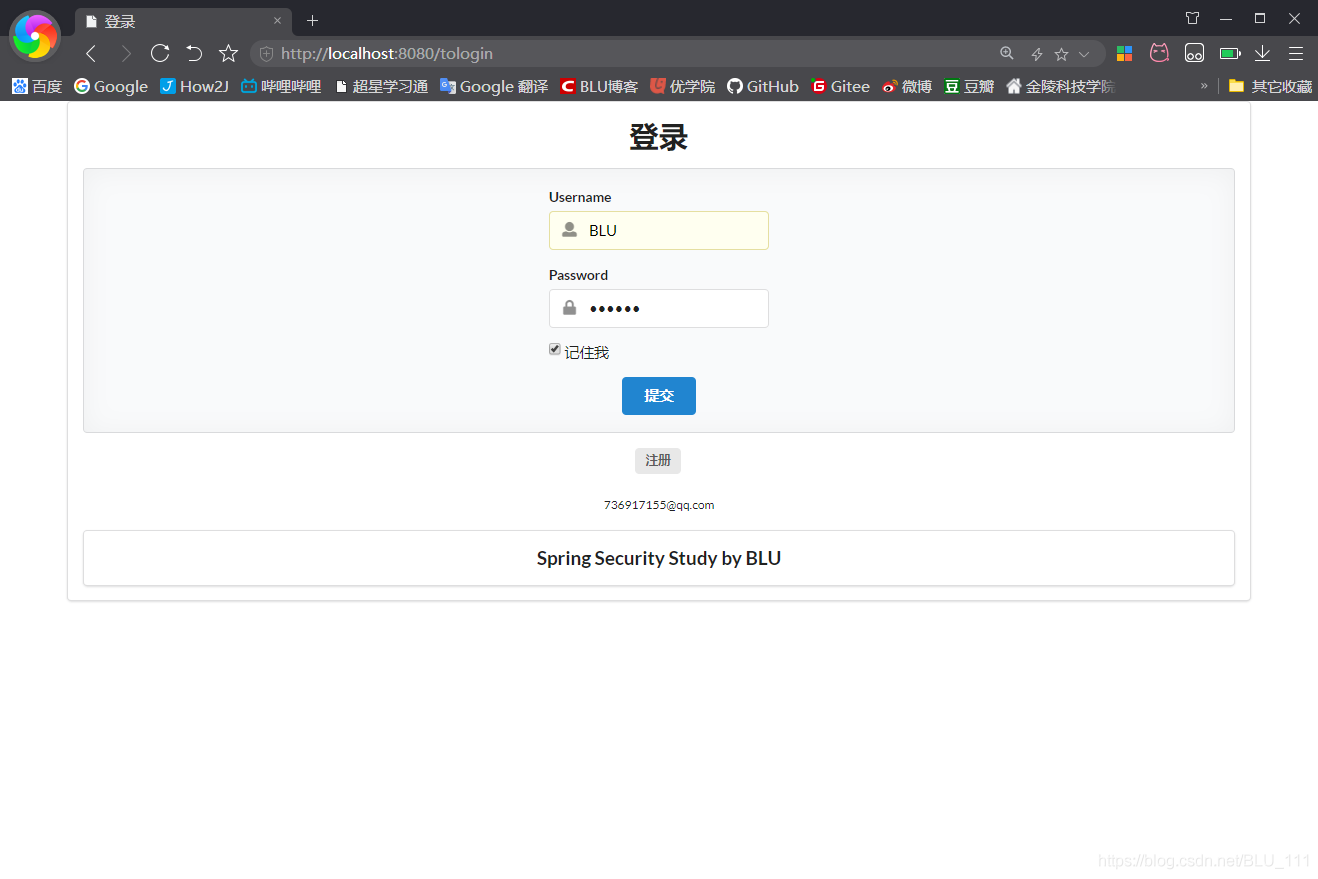
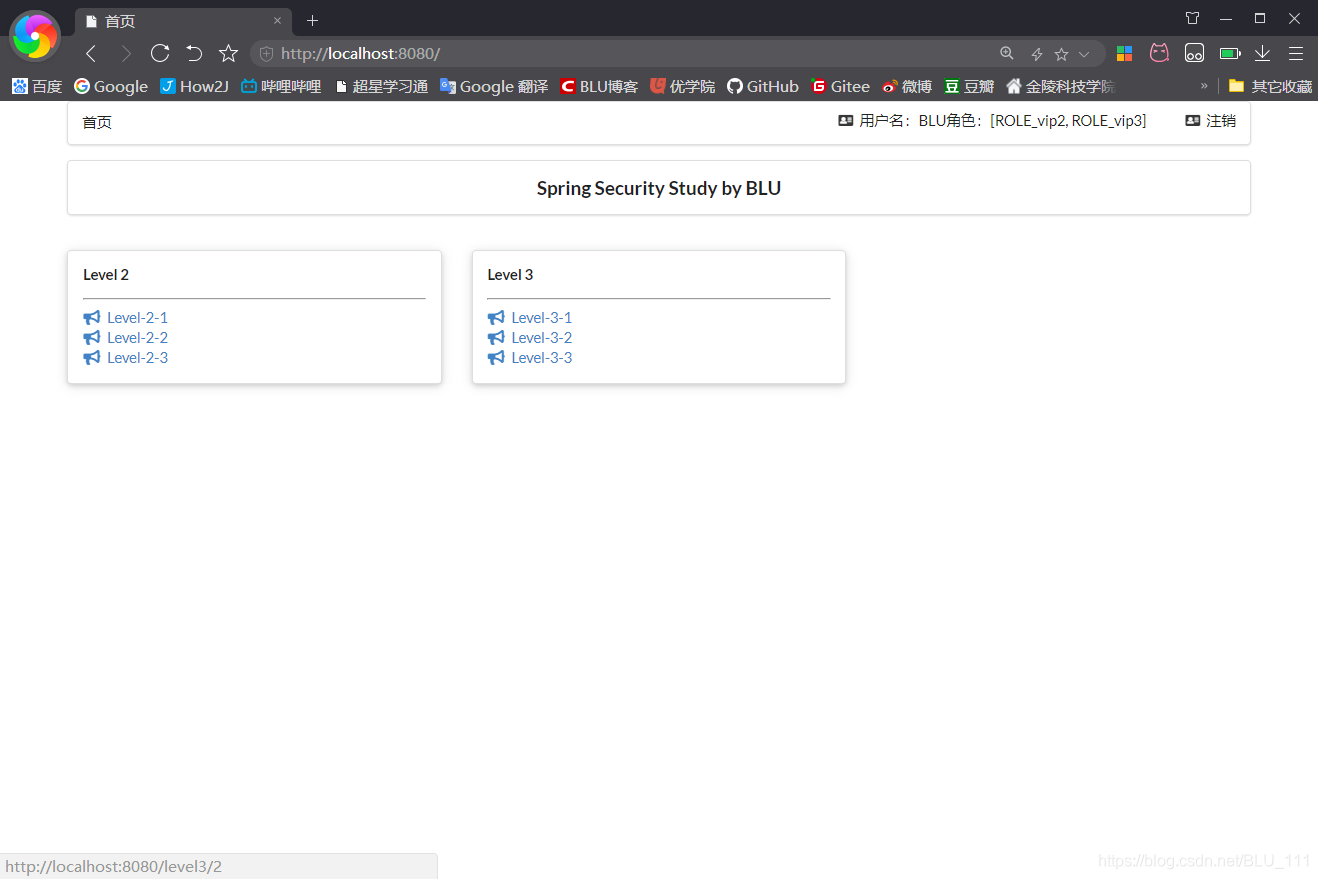
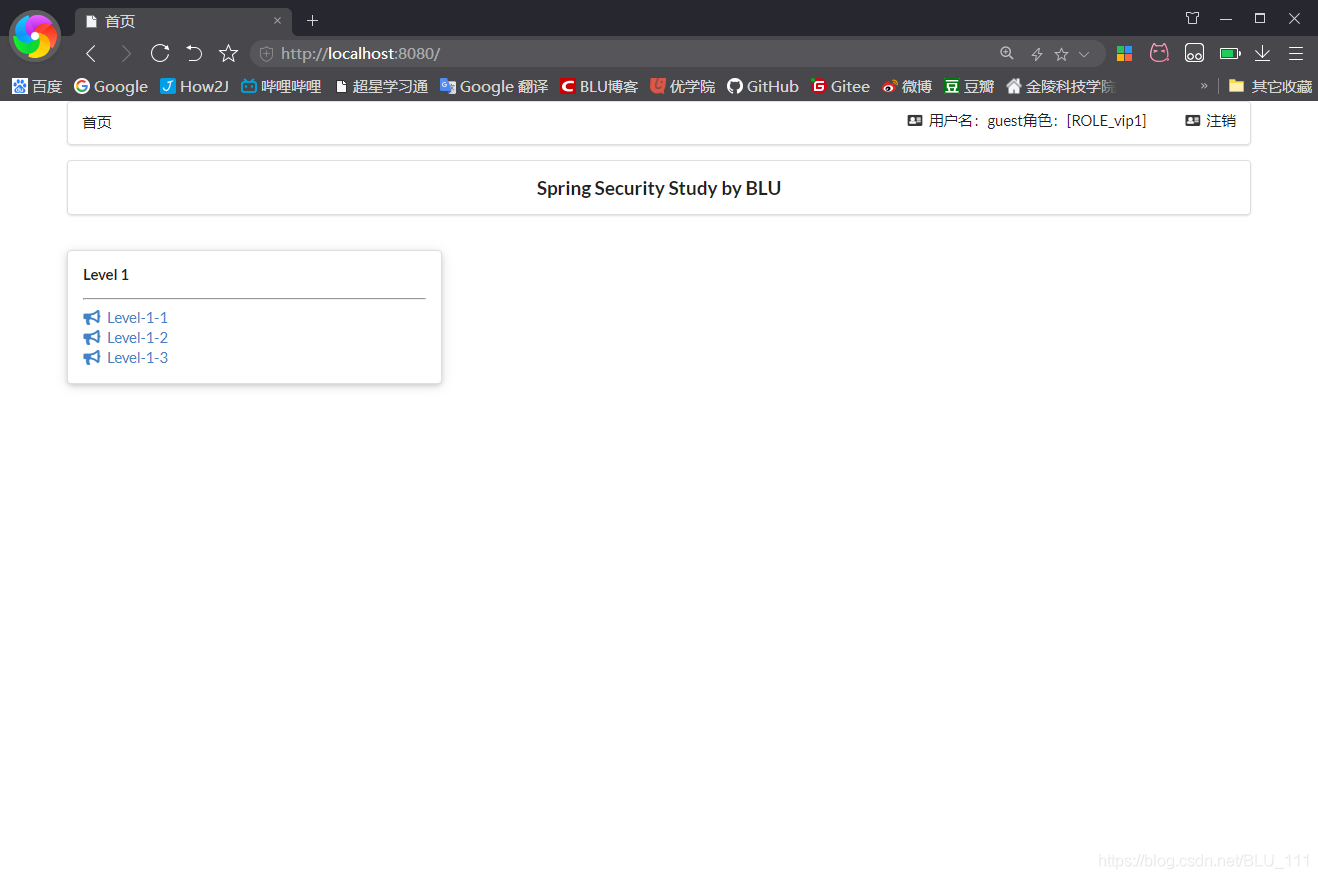
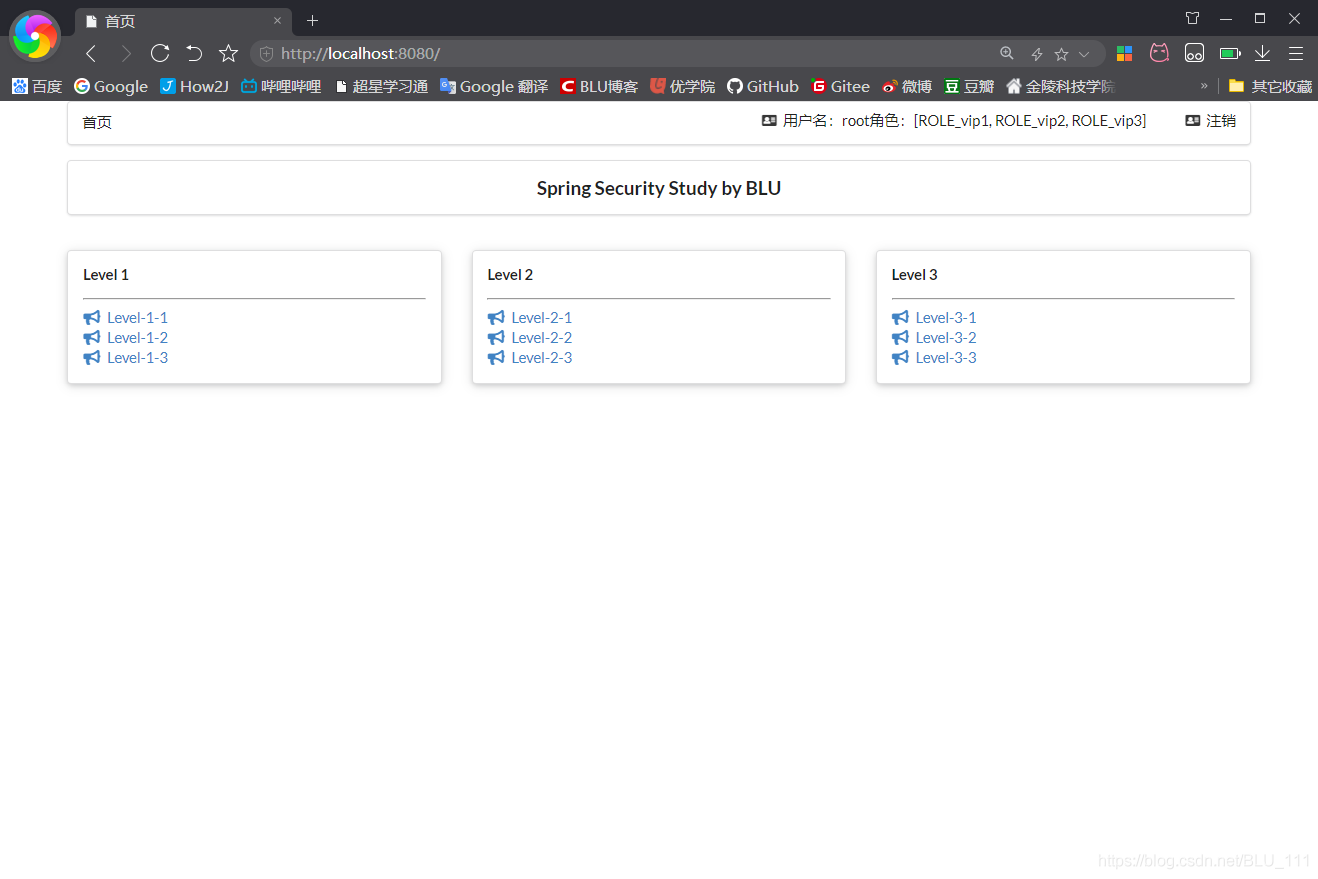
項目源碼:
鏈接: https://pan.baidu.com/s/1AtbcCht84NT-69-sSUAQRw
提取碼: nh92
到此這篇關於SpringBoot與SpringSecurity整合的文章就介紹到這瞭,更多相關SpringBoot與SpringSecurity整合內容請搜索WalkonNet以前的文章或繼續瀏覽下面的相關文章希望大傢以後多多支持WalkonNet!
推薦閱讀:
- 詳解springboot springsecuroty中的註銷和權限控制問題
- Spring Security的簡單使用
- SpringBoot靜態資源CSS等修改後再運行無效的解決
- SpringBoot+SpringSecurity實現基於真實數據的授權認證
- java SpringSecurity使用詳解