Java異常處理實例詳解
1. 異常例子
class TestTryCatch { public static void main(String[] args){ int arr[] = new int[5]; arr[7] = 10; System.out.println("end!!!"); } }
輸出:(越界)
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 7 at TestTryCatch.main(TestTryCatch.java:4) 進程已結束,退出代碼1
2. 異常處理
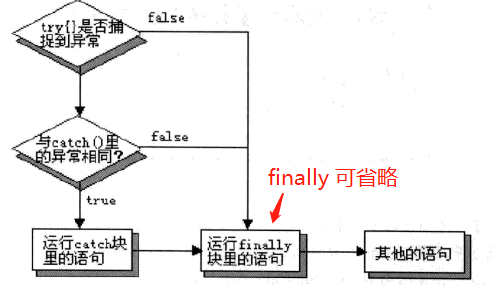
class TestTryCatch { public static void main(String[] args){ try { int arr[] = new int[5]; arr[7] = 10; } catch (ArrayIndexOutOfBoundsException e){ System.out.println("數組范圍越界!"); System.out.println("異常:"+e); } finally { System.out.println("一定會執行finally語句塊"); } System.out.println("end!!!"); } }
輸出:
數組范圍越界! 異常:java.lang.ArrayIndexOutOfBoundsException: 7 一定會執行finally語句塊 end!!!
3. 拋出異常
語法:throw 異常類實例對象;
int a = 5, b = 0; try{ if(b == 0) throw new ArithmeticException("一個算術異常,除數0"); else System.out.println(a+"/"+b+"="+ a/b); } catch(ArithmeticException e){ System.out.println("拋出異常:"+e); }
輸出:
拋出異常:java.lang.ArithmeticException: 一個算術異常,除數0
對方法進行異常拋出
void add(int a, int b) throws Exception { int c = a/b; System.out.println(a+"/"+b+"="+c); }
TestTryCatch obj = new TestTryCatch(); obj.add(4, 0);
輸出:(報錯)
java: 未報告的異常錯誤java.lang.Exception; 必須對其進行捕獲或聲明以便拋出
可見,方法後面跟瞭 throws 異常1, 異常2...
,則 必須 在調用處 處理
改為:
TestTryCatch obj = new TestTryCatch(); try{ obj.add(4, 0); } catch (Exception e){ System.out.println("必須處理異常:"+e); }
輸出:
必須處理異常:java.lang.ArithmeticException: / by zero
4. 編寫異常類
語法:(繼承 extends Exception
類)
class 異常類名 extends Exception{ ...... }
class MyException extends Exception{ public MyException(String msg){ // 調用 Exception 類的構造方法,存入異常信息 super(msg); } }
try{ throw new MyException("自定義異常!"); } catch (Exception e){ System.out.println(e); }
輸出:
MyException: 自定義異常!
到此這篇關於Java異常處理實例詳解的文章就介紹到這瞭,更多相關Java異常處理內容請搜索WalkonNet以前的文章或繼續瀏覽下面的相關文章希望大傢以後多多支持WalkonNet!