Java多線程基礎
一、線程
什麼是線程:
線程是進程的一個實體,是CPU調度和分派的基本單位,它是比進程更小的能獨立運行的基本單位。
什麼是多線程:
多線程指在單個程序中可以同時運行多個不同的線程執行不同的任務。
二、創建多線程的方式
多線程的創建方式有三種:Thread
、Runnable
、Callable
1、繼承Thread類實現多線程
Thread thread = new Thread() { @Override public void run() { super.run(); while (true) { try { Thread.sleep(500); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("1:" + Thread.currentThread().getName()); System.out.println("2:" + this.getName()); } } }; thread.start();
2、實現Runnable接口方式實現多線程
Thread thread1 = new Thread(new Runnable() { @Override public void run() { while (true) { try { Thread.sleep(500); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("3:" + Thread.currentThread().getName()); } } }); thread1.start();
3、Callable接口創建線程
public class CallableTest { public static void main(String[] args) { System.out.println("當前線程是:" + Thread.currentThread()); Callable myCallable = new Callable() { @Override public Integer call() throws Exception { int i = 0; for (; i < 10; i++) { } //當前線程 System.out.println("當前線程是:" + Thread.currentThread() + ":" + i); return i; } }; FutureTask<Integer> fu = new FutureTask<Integer>(myCallable); Thread th = new Thread(fu, "callable thread"); th.start(); //得到返回值 try { System.out.println("返回值是:" + fu.get()); } catch (Exception e) { e.printStackTrace(); } } }
當前線程是:Thread[main,5,main]
當前線程是:Thread[callable thread,5,main]:10
返回值是:10
總結:
實現Runnable
接口相比繼承Thread類有如下優勢:
- 可以避免由於Java的單繼承特性而帶來的局限;
- 增強程序的健壯性,代碼能夠被多個線程共享,代碼與數據是獨立的;
- 適合多個相同程序代碼的線程區處理同一資源的情況。
實現Runnable
接口和實現Callable
接口的區別:
Runnable
是自從java1.1
就有瞭,而Callable
是1.5之後才加上去的Callable
規定的方法是call()
,Runnable
規定的方法是run()
Callable
的任務執行後可返回值,而Runnable
的任務是不能返回值是(void)- call方法可以拋出異常,run方法不可以
- 運行Callable任務可以拿到一個Future對象,表示異步計算的結果。它提供瞭檢查計算是否完成的方法,以等待計算的完成,並檢索計算的結果。通過Future對象可以瞭解任務執行情況,可取消任務的執行,還可獲取執行結果。
- 加入線程池運行,
Runnable
使用ExecutorService
的execute
方法,Callable
使用submit
方法。
三、線程的生命周期與狀態
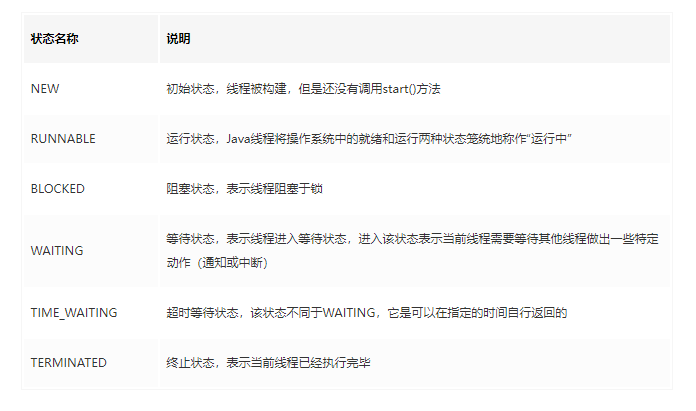
四、線程的執行順序
Join
線程的運行順序
原理:
1、定時器
import java.util.Timer; import java.util.TimerTask; public class TraditionalTimerTest { public static void main(String[] args) { // new Timer().schedule(new TimerTask() { // @Override // public void run() { // // System.out.println("bombing!"); // } // },10000); class MyTimberTask extends TimerTask { @Override public void run() { System.out.println("bombing!"); new Timer().schedule(new MyTimberTask(), 2000); } } new Timer().schedule(new MyTimberTask(), 2000); int count = 0; while (true) { System.out.println(count++); try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } } }
0
1
bombing!
2
3
bombing!
4
5
bombing!
6
省略…
2、線程的互斥與同步通信
public class TraditionalThreadSynchronized { public static void main(String[] args) { new TraditionalThreadSynchronized().init(); } private void init() { final Outputer outputer = new Outputer(); new Thread(new Runnable() { @Override public void run() { while (true) { try { Thread.sleep(10); } catch (InterruptedException e) { e.printStackTrace(); } outputer.output("kpioneer"); } } }).start(); // new Thread(new Runnable() { // @Override // public void run() { // // while (true) { // try { // Thread.sleep(10); // } catch (InterruptedException e) { // e.printStackTrace(); // } // outputer.output2("Tom"); // // } // } // }).start(); new Thread(new Runnable() { @Override public void run() { while (true) { try { Thread.sleep(10); } catch (InterruptedException e) { e.printStackTrace(); } outputer.output3("Jack"); } } }).start(); } static class Outputer { public void output(String name) { int len = name.length(); synchronized (Outputer.class) { for (int i = 0; i < len; i++) { System.out.print(name.charAt(i)); } System.out.println(); } } public synchronized void output2(String name) { int len = name.length(); for (int i = 0; i < len; i++) { System.out.print(name.charAt(i)); } System.out.println(); } public static synchronized void output3(String name) { int len = name.length(); for (int i = 0; i < len; i++) { System.out.print(name.charAt(i)); } System.out.println(); } } }
3、線程同步通信技術
子線程循環10次,接著主線程循環100,接著又回到子線程循環10次,接著再回到主線程有循環100,如此循環50次,請寫出程序。
public class TraditionalThreadCommunication { public static void main(String[] args) { final Business business = new Business(); new Thread(new Runnable() { @Override public void run() { for (int i = 1; i <= 50; i++) { business.sub(i); } } } ).start(); for (int i = 1; i <= 50; i++) { business.main(i); } } } /** *要用到共同數據(包括同步鎖)的若幹方法應該歸在同一個類身上, * 這種設計正好體現瞭高類聚和和程序的健壯性 */ class Business { private boolean bShouldSub = true; public synchronized void sub(int i) { if(!bShouldSub) { try { this.wait(); } catch (InterruptedException e) { e.printStackTrace(); } } for (int j = 1; j <= 10; j++) { System.out.println("sub thread sequece of " + j + ",loop of " + i); } bShouldSub = false; this.notify(); } public synchronized void main(int i) { if(bShouldSub) { try { this.wait(); } catch (InterruptedException e) { e.printStackTrace(); } } for (int j = 1; j <=100; j++) { System.out.println("main thread sequece of " + j + ",loop of " + i); } bShouldSub = true; this.notify(); } }
sub thread sequece of 1,loop of 1
sub thread sequece of 2,loop of 1
sub thread sequece of 3,loop of 1
sub thread sequece of 4,loop of 1
sub thread sequece of 5,loop of 1
sub thread sequece of 6,loop of 1
sub thread sequece of 7,loop of 1
sub thread sequece of 8,loop of 1
sub thread sequece of 9,loop of 1
sub thread sequece of 10,loop of 1
main thread sequece of 1,loop of 1
main thread sequece of 2,loop of 1
main thread sequece of 3,loop of 1
main thread sequece of 4,loop of 1
main thread sequece of 5,loop of 1
main thread sequece of 6,loop of 1
main thread sequece of 7,loop of 1
main thread sequece of 8,loop of 1
main thread sequece of 9,loop of 1
main thread sequece of 10,loop of 1
main thread sequece of 11,loop of 1
省略中間…
main thread sequece of 99,loop of 1
main thread sequece of 100,loop of 1
sub thread sequece of 1,loop of 2
sub thread sequece of 2,loop of 2
sub thread sequece of 3,loop of 2
sub thread sequece of 4,loop of 2
sub thread sequece of 5,loop of 2
sub thread sequece of 6,loop of 2
sub thread sequece of 7,loop of 2
sub thread sequece of 8,loop of 2
sub thread sequece of 9,loop of 2
sub thread sequece of 10,loop of 2
main thread sequece of 1,loop of 2
main thread sequece of 2,loop of 2
main thread sequece of 3,loop of 2
省略…
到此這篇關於Java多線程基礎的文章就介紹到這瞭,更多相關Java多線程內容請搜索WalkonNet以前的文章或繼續瀏覽下面的相關文章希望大傢以後多多支持WalkonNet!